CSE1OOF/CSE4OOF Assessment
Create an object-oriented design from a problem description,
and implement as a working Java program
Assigned to students 4th October 2021
Due Sunday 24 October @ 11:55pm (end of Week 13)
Worth 20% of your final marks No late submissions accepted
You will, using object-oriented principles, design a set of interrelated classes and implement them as a Java program. It’s your final grand challenge!
Specifically, you will create and document a program for organizing AFL team members, teams, and results.
Part I: AFLTeamMember (10 marks)
You will implement a class AFLTeamMember. Each team member (players, coaches, etc.) has a
name and a position.
The positions on an AFL team are as follows: FB, HB, C, HF, FF, FOL, IC, COACH.
The String representation of an AFLTeamMember should be as follows: “FirstName LastName, POSITION”. For example, “Simon Goodwin, COACH”.
You will implement this class, including getter and setter methods as appropriate. Part II: AFLPlayer (10 marks)
You will implement a class AFLPlayer. This will be a child class of AFLTeamMember. Each AFLPlayer, in addition to having a name and a position, will also have a number, and may or may notbe a captain.
The String representation of an AFLPlayer should be as follows “[Number] FirstName Last
Name, POSITION”, followed by “(c)” if that player is a captain. For example, “[1] Adam Treloar, HF”, or “[11] Max Gawn, FOL (c)”.
You will implement this class, including getter and setter methods as appropriate. Part III: AFLTeam (10 marks)
You will implement a class AFLTeam. Each team has a name, a coach, and a lineup consisting of
22 players.
You will implement this class, including getter and setter methods as appropriate. Part IV: AFLMatch (10 marks)
You will implement a class AFLMatch. An AFL match has a homeTeam, an awayTeam, and the scores for each team. In an AFL match, each score can either be a goal, worth 6 points, or a behind, worth 1 point. You will need to keep track of these separately, as in the number of homeGoals, the number of homeBehinds, the number of awayGoals, and the number of awayBehinds. This class will also contain the main() method for this program.
You will implement this class, including getter and setter methods as appropriate.
Part V: Load in the lineups (20 marks)
To load the lineups of the two teams, you will accept as input at the command line the names of two files. The first filename will be the name of a file the home team’s lineup, and the second filename will be the name of a file containing the away team’s lineup.
For example, you would start the program as:
> java AFLMatch MelbourneDemons.txt WesternBulldogs.txt
This would load the home team’s lineup from MelbourneDemons.txt, and the away
team’s lineup from WesternBulldogs.txt. These two files are provided to you for testing; the content of WesternBulldogs.txt is included here:
Western Bulldogs
Luke Beveridge, COACH 10, Easton Wood, FB 42, Alex Keath, FB
15, Taylor Duryea, FB 35, Caleb Daniel, HB 12, Zaine Cordy, HB 31, Bailey Dale, HB
6, Bailey Smith, C
21, Tom Liberatore, C 7, Lachie Hunter, C
19, Cody Weightman, HF 33, Aaron Naughton, HF 1, Adam Treloar, HF
39, Jason Johannisen, FF 44, Tim English, FF
29, Mitch Hannan, FF 8, Stefan Martin, FOL 11, Jack Macrae, FOL
4, Marcus Bontempelli, FOL, c 34, Bailey Williams, IC
5, Josh Dunkley, IC 37, Roarke Smith, IC 13, Josh Schache, IC
Part VI: Run the game (20 marks)
You will use a loop to take input from the user, who is the “scorekeeper.” This loop will prompt the user to type one letter to indicate which team scored: “h” for the home team or “a” for the away team. It will then prompt the user to type one letter to indicate the type of score: “g” for goal or “b” for behind. This loop will keep running until the user types “f”, to indicate full time (the end of the match). In action, the loop might look like this:
|
Which team scored? h Goal or behind? b
Melbourne Demons 1.1 (7) defeated Western Bulldogs 1.0 (6)
Part VII: Add exceptions and exception handling (20 marks)
At this point, you have a program that “works”, but isn’t really complete. For example, you could put in a negative number for a player’s number, or you could list someone at a position
other than COACH as the team’s coach, or your lineup can have more or less than 22 players. All of these should be illegal, but our program doesn’t handle any of these cases yet. These are all examples of run-time problems, which should throw exceptions when they occur.
You will modify your program to define appropriate Exception types, throw them at appropriate types, and handle them in appropriate places in your code. Specifically, you should check for the following problems in your program:
- A filename that is invalid (does not correspond to an existing file of the appropriate format)
- A player who has a number that is invalid (negative or not an integer)
- A team member who has an invalid position
- A team that has more or fewer than eighteen players
- A team that has more or fewer than one captain
- A value other than “h”, “a”, “b”, “g”, or “f” is entered in the scorekeeping loop
Expert's Answer
Chat with our Experts
Want to contact us directly? No Problem. We are always here for you
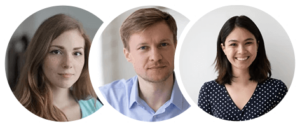
Get Online
Online Tutoring Services